Using Azure Key Vault secrets in PowerShell scripting
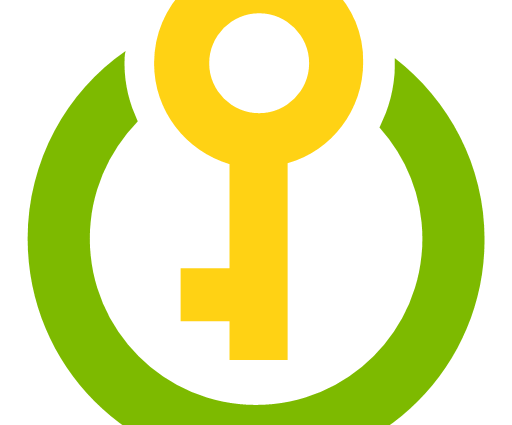
I've been writing a lot of scripts lately and one of the things I come across often is the way people store credentials in a PowerShell script. Often they are either hardcoded (and people forget about them) or they are fetched from a text file. The last option is prompting for each passwords.
Now for a script that uses multiple passwords a prompt isn't really the way you want to deal with this. As most of the scripts I'm writing (if not all) are performing actions in Azure, chances are near 100% that I'm already logged into Azure PowerShell. Why not leverage Azure Key Vault?
The Script
The script I'm using is relatively straight forward. I'm connecting to my Azure Key Vault, grab all the secrets and store them in a table. Afterwards I'll be able to address them whenever I need them.
All it takes is you to be logged into to Azure (Login-AzAccount) and run the code.
$keyvaultName = 'KeyVaultName'
$secrets = Get-AzKeyVaultSecret -VaultName $keyvaultName
$keys =@{}
foreach ($secret in $secrets)
{
$secretName = $secret.name
$key = (Get-AzKeyVaultSecret -VaultName $keyvaultName -name $secretName).SecretValueText
$keys.Add("$secretName", "$key")
}
Every secret stored in your Key Vault is now available through the $keys table like so:
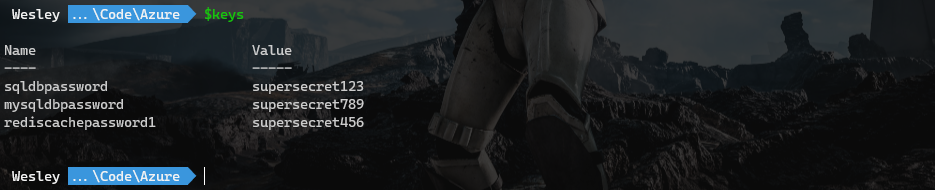
By referencing a specific secret you can retrieve the value and use the secret in your script (for instance during a deployment).

When the script is gone, the table is gone. No more storing passwords in your scripts!